Announced at PDC this week
http://www.microsoft.com/downloads/details.aspx?FamilyID=6806e466-dd25-482b-a9b3-3f93d2599699&displaylang=en
Finally a version of blend that will open vs2010 projects (and of course handle Wpf4 and Silverlight 4)
It will run side by side with Blend 3
Enjoy 
It's true what they say, to learn new technologies it's simple a matter of ABC (Always be coding), I've watched many the video, read many the book but it's only when I went to try to do something the it sinks in.
Like tonight while trying to host a WCF service on my Windows 7 machine I came across a problem that you'd only find by ABC.
HTTP Error 404.3 - Not Found
The page you are requesting cannot be served because of the extension configuration. If the page is a script, add a handler. If the file should be downloaded, add a MIME map.
The solution.
Control Panel/Programs and Features/Add or Remove components/Microsoft .NET Framework 3.5.1 section.
I checked the two Windows Communication Foundation checkboxes shown below (the second box enables Windows Activation Service - WAS so that IIS can host WCF services that can be called using non-HTTP bindings such as TCP) (nice!)
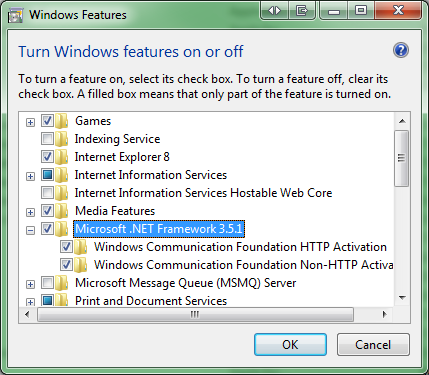
http://errorlist.codeplex.com/
ErrorList
I recently wrote a control that tries to replicate the error list seen in Visual studio.
The control is dependant on .NET 3.5 SP1. because it uses the WPFToolkit.
I'm hosting a sample here. [Update August 2012 My host has stopped xbap application types, sorry]
Screenshot
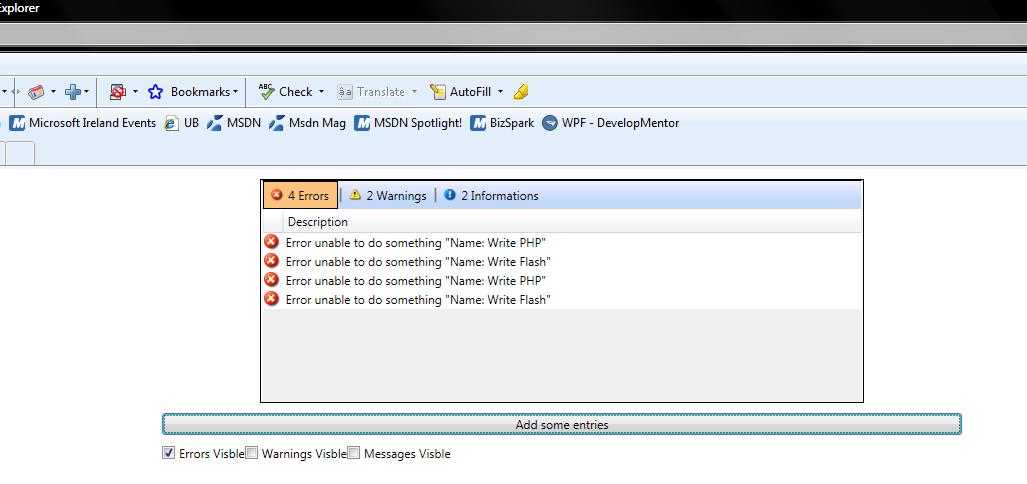
I've had a few people asking me how did I host Silverlight 3 in blog engine.
Silverlight Usercontrol
It was pretty simple really (when you know how)
Basically Silverlight3 needs an <object> tag.
So I created a user control with the object tag and some script handling for client side errors (actually VS2010 did all the hard lifting).
[code:c#]
<%@ Control Language="C#" ClassName="SilverlightControl" %>
<script runat="server">
</script>
<script type="text/javascript" src="~/Silverlight.js"></script>
<script type="text/javascript">
function onSilverlightError(sender, args) {
var appSource = "";
if (sender != null && sender != 0) {
appSource = sender.getHost().Source;
}
var errorType = args.ErrorType;
var iErrorCode = args.ErrorCode;
if (errorType == "ImageError" || errorType == "MediaError") {
return;
}
var errMsg = "Unhandled Error in Silverlight Application " + appSource + "\n";
errMsg += "Code: " + iErrorCode + " \n";
errMsg += "Category: " + errorType + " \n";
errMsg += "Message: " + args.ErrorMessage + " \n";
if (errorType == "ParserError") {
errMsg += "File: " + args.xamlFile + " \n";
errMsg += "Line: " + args.lineNumber + " \n";
errMsg += "Position: " + args.charPosition + " \n";
}
else if (errorType == "RuntimeError") {
if (args.lineNumber != 0) {
errMsg += "Line: " + args.lineNumber + " \n";
errMsg += "Position: " + args.charPosition + " \n";
}
errMsg += "MethodName: " + args.methodName + " \n";
}
throw new Error(errMsg);
}
</script>
<div id="silverlightControlHost">
<object data="data:application/x-silverlight-2," type="application/x-silverlight-2" width="100%" height="100%">
<param name="source" value="<%Silverlight.xbap%>"/> // where <%Silverlight.xbap%> is location of silverlight xbap
<param name="onError" value="onSilverlightError" />
<param name="background" value="white" />
<param name="minRuntimeVersion" value="3.0.40818.0" />
<param name="autoUpgrade" value="true" />
<a href="http://go.microsoft.com/fwlink/?LinkID=149156&v=3.0.40818.0" style="text-decoration:none">
<img src="http://go.microsoft.com/fwlink/?LinkId=161376" alt="Get Microsoft Silverlight" style="border-style:none"/>
</a>
</object><iframe id="_sl_historyFrame" style="visibility:hidden;height:0px;width:0px;border:0px"></iframe></div>
[/code]
One approach for validating child controls on windows froms is to
Validation.
- Add a Validated event handler to all child controls you're interested in.
- On a button event handler call this.ValidateChildren();
This will ensure the validation routine on all child controls will be called, if for example you've added an ErrorProvider control extender to your form you can set it up in the validated event handlers.
A quick example of inline xml and Linq
Code
[code:c#]
void LinqToXmlSample()
{
Console.WriteLine("{0} : Start", new StackTrace(0, true).GetFrame(0).GetMethod().Name);
XDocument xDocument = new XDocument(
new XElement("people",
new XElement("person",
new XAttribute("sex", "male"),
new XElement("FirstName", "Brian"),
new XElement("LastName", "Keating")),
new XElement("person",
new XAttribute("type", "female"),
new XElement("FirstName", "Dustin"),
new XElement("LastName", "Turkey"))));
IEnumerable<XElement> elements =
xDocument.Element("people").Descendants("FirstName");
/* First, I will display the source elements.*/
foreach (XElement element in elements)
{
Console.WriteLine("Source element: {0} : value = {1}",
element.Name, element.Value);
}
/* Now, I will display the ancestor elements for each source element.*/
foreach (XElement element in elements.Ancestors())
{
Console.WriteLine("Ancestor element: {0}", element.Name);
}
Console.WriteLine("{0} : Finish", new StackTrace(0, true).GetFrame(0).GetMethod().Name);
}
[/code]
Output
LinqToXmlSample : Start
Source element: FirstName : value = Brian
Source element: FirstName : value = Dustin
Ancestor element: person
Ancestor element: people
Ancestor element: person
Ancestor element: people
LinqToXmlSample : Finish
[Update August 2012: Little did I know when i wrote this post just how much I would use Linq to xml, it saved me from a life of xslt
]
A neat way of always cleaning up resources is to use Lambdas as data.
Take the following
Source
[code:c#]
internal interface ITryCatchReport
{
void Try(Action<IServer> action);
}
internal class TryCatchReport : ITryCatchReport
{
public TryCatchReport(IServer server)
{
_server = server;
}
public void Try(Action<IServer> action)
{
try
{
action(_server);
}
catch (Exception e)
{
Trace.WriteLine(e.Message);
// Clean up resources
// Report errors
}
}
private IServer _server;
}
[/code]
Usage
[code:c#]
TryCatchReport safeInvoker = new TryCatchReport(_data.Server);
safeInvoker.Try(x =>
{
x.MakeInterfaceCall();
});
[/code]
We are now guaranteed that in the case of an exception that the resources will get cleaned up.
Usage with code blocks
If you wish to execute many statements in the action look at this sample.
[code:c#]
private List<WFActionDefinition> GetActionDefinitions()
{
if (_actionDefinitions == null)
{
safeInvoker.Try(x =>
{
x.Do1();
x.DoSomething();
OtherFunc();
});
}
return _actionDefinitions;
}
[/code]
Adding project dependencies on Visual studio is done automatically with .NET projects when Add Reference dialog chooses another project from your solution.
However you can get Visual Studio to add project dependencies if no explicit intra project reference exists.
Right click on your solution, choose properties and choose the project dependencies option.
Screenshot
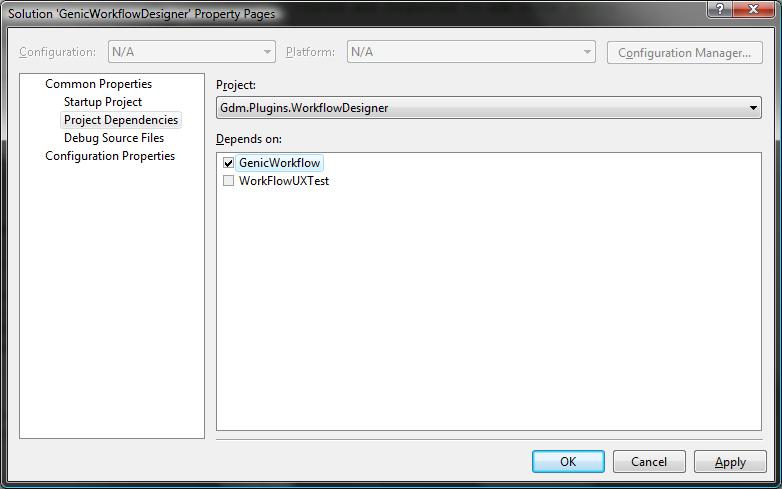
Here's a video from Steve White, a program manager in expression blend.
He shows how to Style a button in Silverlight using expression blend 3.
http://electricbeach.org/apps/Silverlight Button Control Styling Tips/Default.html
Brian.
Ok, forget what you learned about workflow in 3.5.
Windows Workflow 4.0
4.0 changes everything you thought you knew about workflow.
WF 4.0 represents a bottom-up rewrite with entirely new thinking. The gains are enormous: custom activities take centre stage, and authoring them is much simpler; workflows are entirely declarative; and there are three workflow flow styles that you can combine seamlessly. It's possible that you could see a 10-fold improvement in the time required to create and debug workflows, in addition to 10- to 100-fold runtime performance improvements. You'd also have better control over persistence. Additionally, there's support for partial trust, a completely rewritten designer, and a better debugging experience. One of the most important improvements is in the re-hosting experience. This allows you to create activities and to allow power users -- and others -- to build or modify workflows with the constrained set of activities you've created. Yes, this is possible in WF 3.0/3.5, but the necessary code is challenging, not to mention exceedingly difficult to debug.
The improvements to WF 4.0 come at a cost. Microsoft developed two inter-op strategies to help you over this hurdle. WF 3.0/3.5 will remain part of the framework and will run side by side with WF 4.0. This lets you manage the transition at a time that fits your organization's broader goals. The other inter-op strategy is an inter-op activity that allows you to use WF 3.0/3.5 activities in a WF 4.0 workflow. Workflows are a specialization of activities, so you'll probably be able to run entire 3.0/3.5 workflows inside WF 4.0 workflows.
Screenshot VS2010 B2
